You've set up your Rails app and want to send out emails to your users, for example welcome emails for sign ups or thank you emails when they completed an order.
In order to do that you'll need Rails' ActionMailer class and a third party email service, for example SendGrid.
In the following is an example of how we set it up for our FlatironShop website.
First of, you create a Mailer controller by inheriting from ActionMailer::Base and put the file in 'app/mailers'.
This is an example for FlatironShop, where we specify a method called order_email:
Like a controller, UserMailer needs a view to render. So we need to create order_email.html.erb which will serve as the template:

Now you can use the ActionMailer methods in your controllers to render the view, i.e. send the email, just like any other method. We are calling our order_email method on the UserMailer class that we created above, pass in the arguments and call 'deliver_now'
This is how it looks like in the orders_controller:

And that's it for the MVC part.
Now, assuming that your app is deployed with Heroku, we need to set up a SendGrid account so that we can retrieve the API key and set the ActionMailer's SMTP settings to use the SendGrid credentials.
After you've set up your account (you can choose the free version) run in the console:
To get the API key, log into your SendGrid account, click the “Create API Key” button, and a dropdown menu will appear allowing you to choose the type of API Key you would like to create.

Set the environment in the console:
Now that the API key has been set we need to include them in the Gemfile (don't forget to run 'bundle'):
The last step is to configure the ActionMailer to use SendGrid:
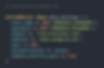
And voila! That's it. Try it out!
Happy emailing!
There is great documentation for ActionMailer and SendGrid:
ActionMailer:
http://guides.rubyonrails.org/action_mailer_basics.html
SendGrid with Heroku:
https://devcenter.heroku.com/articles/sendgrid